Java throw keyword is used to throw an exception explicitly (manually). The throw keyword must be in try block. When JVM encounters the throw keyword, it stops the execution of try block and jump to the corrosponding catch block. COMPUTER PROGRAMMING WITH JAVA Page 41 black white d.printColor; Output: Final Keyword in Java The final keyword in java is used to restrict the user. The java final keyword can be used in many context. Final can be: 1. Class The final keyword can be applied with the variables, a final variable that have no value it is called blank final variable. We will discuss the below keywords in a separate tutorial as they have a great significance as far as Java programming is concerned. These words are: #1) “this” keyword. The keyword “this” points to the current object in the program. Also Read = Java ‘THIS’ Keyword With Code Examples #2) “static” keyword. Here, int is a keyword. It indicates that the variable score is of integer type (32-bit signed two's complement integer). You cannot use keywords like int, for, class, etc as variable name (or identifiers) as they are part of the Java programming language syntax. Here's the complete list of all keywords in Java programming.
Useful Java Terms
Some definitions taken from:
http://java.about.com/od/beginningjava/a/javaglossary.htm
http://java.sun.com/docs/glossary.html
Assign – to set the data of a variable (involves the equal sign).
Example 1: assign the value 2 to the integer var.
int var = 2;
Example 2:
// myObject1 is assigned a new instance of MyObject().
MyClass myObject1 =new MyObject();
// myObject2 is assigned the same instance as myObject1
MyClass myObject2 = myObject1;
Class - A class describes a particular kind of object. It can contain related methods and data members (variables). A class must have the same name as the file it is contained in.
Example:
publicclass myClass(){
//data members
//constructors
//methods
}
Constructor - A special type of instance method that creates a new object. In Java, constructors have the same name as their class and have no return value in their declaration.
Keywords In Java Language
Example:
publicclass myClass{
// a constructor that takes no parameters
public myClass(){
}
// a constructor that takes one parameter
public myClass(int var){
}
}
Declaration - A statement that creates a variable, method, or class identifier and its associated attributes but doesn't necessarily allocate storage for variables or define an implementation for methods. Classes are always defined when they are declared, i.e., a class cannot be declared and then have its body defined elsewhere.
Example 1: the variable var is declared
int var;
Example 2: the object myObject is declared as an instance of the MyClass class.
MyClass myObject;
Example 3: abstract methods, found in abstract classes, can be declared but not defined.
publicabstractclass myAbstractClass(){
// abstract methods are declared.
publicabstract myMethod()
}
Example 4: methods found in interfaces can be declared but not defined.

publicinterface myInterface(){
// methods in an interface are declared
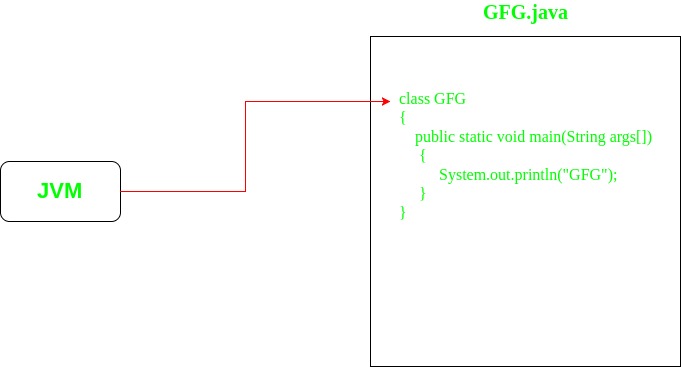
publicvoid myMethod();
}
Definition – Similar to a declaration except that it also reserves storage (for variables) or provides implementations (for methods).
Example 1: variables created from primitive data types are defined when declared.
// this declaration also allocates storage for var.
int var;
Example 2: a method is defined if it has brackets, a space where code goes that says what the method does.
publicvoid myMethod(){
/* these brackets can contain code that defines what this method does */
}
Garbage Collection – Programs require memory to run. Memory for objects is allocated by the keyword new. When objects are no longer used or your program terminates, Java automatically frees the used memory for other uses.
Initialize – an assignment that sets the starting value of a variable.
Example 1: an integer var is declared, defined, and initialized to the value 2.
int var = 2;
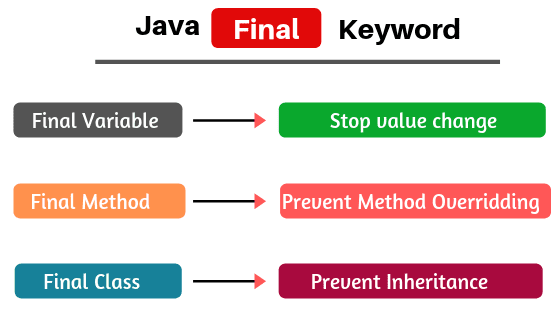
Example 2: an integer var is declared and defined. Then it is initialized to 2.
int var;
var = 2;
Example 3: an object is declared via “MyClass myObject”, instantiated via “new”, and initialized by calling its constructor, “MyClass().”
MyClass myObject = new MyClass(/*parameters*/);
Instantiate – To allocate storage for an object in memory (involves the keyword new).
Example: the keyword new instantiates the object below. An instantiation is always followed by a constructor call that initializes the object.
MyClass myObject = new MyClass(/*parameters*/);
Method – a collection of code found within a class. If the data members of a class are nouns, the methods are the verbs (the action).
Example 1: a method, myMethod is defined within class myClass that does NOT return a value.
Abstract Keyword In Java Program
publicclass myClass{
publicvoid myMethod(){
}
}
Example 2: a method, myMethod is defined within class myClass that returns a boolean.
publicclass myClass{
publicboolean myMethod(){
returntrue;
}
}
Object - The principal code building block of Java programs. Each object in a program consists of both variables (data) and methods (functionality).
Parameter – a variable or object passed into a method.

Example 1: a method, myMethod is defined which takes 2 parameters.
publicclass MyClass{
publicvoid myMethod(int var1, double var2){
}
}
Example 2: myMethod is called.
int myInteger = 2;
double myDouble = 5.0;
myMethod(myInteger, myDouble);
Primitive – A variable defined with a primitive data type:byte, short, int, long, float, double, char, or boolean.
Typecast – (1) to demote a variable from a larger capacity data type to a smaller one. (2) to re-establish the class of an object. The cast associates itself with the expression to its immediate right.
Example 1: A double var is created and then typecast via “(int)” to a variable of integer type ans.
double var = 3;
int ans = (int) var;
Example 2: All objects extend the Java Object class.
// define class MyClass in file MyClass.java
publicclassMyClass{}
// in the main method of another class…
// instantiate MyClass, but store it in a variable of type Object.
Object o = new MyClass();
// typecast object o, back into an instance of MyClass.
MyClass myObject = (MyClass) o;
Use/Read – the use of a variable in the right hand side of an assignment statement.
Example: an integer var is declared and used in the initialization of integer ans.
int var = 2;
int ans = Math.pow(var,2);
